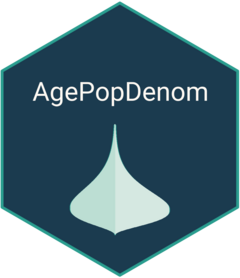
Generate or Load Cached Predictors Data
create_prediction_data.Rd
This function creates predictors data based on spatial inputs or loads cached predictors data if the file already exists. It saves the generated data to a specified directory for reuse and provides progress updates.
Usage
create_prediction_data(
country_code,
country_shape,
pop_raster,
ur_raster,
adm2_shape,
cell_size = 5000,
ignore_cache = FALSE,
output_dir = here::here("03_outputs", "3a_model_outputs")
)
Arguments
- country_code
A string representing the country code (e.g., "KEN").
- country_shape
An `sf` object representing the country's administrative boundaries.
- pop_raster
A `terra` raster object representing the population raster.
- ur_raster
A `terra` raster object representing the urban extent raster.
- adm2_shape
An `sf` object representing the administrative level 2 boundaries.
- cell_size
An integer specifying the cell size for the prediction grid in meters (default is 5000).
- ignore_cache
A boolean input which is set to determine whether to ignore the existing cache and write over it. Default is set to FALSE.
- output_dir
A string specifying the directory where the predictors data file should be saved (default is "03_outputs/3a_model_outputs").
Examples
# \donttest{
tf <- file.path(tempdir(), "test_env")
# Initialize with normalized path
dir.create(tf, recursive = TRUE, showWarnings = FALSE)
init(
r_script_name = "full_pipeline.R",
cpp_script_name = "model.cpp",
path = tf,
open_r_script = FALSE
)
#>
#> ── Package Installation Required ──
#>
#> The following packages are missing:
#> 1. remotes
#> ! Non-interactive session detected. Skipping package installation.
#> ℹ Created: /tmp/RtmpioKZmp/test_env/01_data/1a_survey_data/processed
#> ℹ Created: /tmp/RtmpioKZmp/test_env/01_data/1a_survey_data/raw
#> ℹ Created: /tmp/RtmpioKZmp/test_env/01_data/1b_rasters/urban_extent
#> ℹ Created: /tmp/RtmpioKZmp/test_env/01_data/1b_rasters/pop_raster
#> ℹ Created: /tmp/RtmpioKZmp/test_env/01_data/1c_shapefiles
#> ℹ Created: /tmp/RtmpioKZmp/test_env/02_scripts
#> ℹ Created: /tmp/RtmpioKZmp/test_env/03_outputs/3a_model_outputs
#> ℹ Created: /tmp/RtmpioKZmp/test_env/03_outputs/3b_visualizations
#> ℹ Created: /tmp/RtmpioKZmp/test_env/03_outputs/3c_table_outputs
#> ℹ Created: /tmp/RtmpioKZmp/test_env/03_outputs/3d_compiled_results
#> ✔ Folder structure created successfully.
#> ℹ R script created but could not open automatically: RStudio not available.
#> ✔ C++ script '/tmp/RtmpioKZmp/test_env/02_scripts/model.cpp' successfully created.
# Download shapefiles
download_shapefile(
country_codes = "COM",
dest_file = file.path(
tf, "01_data", "1c_shapefiles",
"district_shape.gpkg"
)
)
#> ℹ Downloading missing WHO ADM2 data for: COM
#> ✔ Created new shapefile with country codes: COM
# Download population rasters from worldpop
download_pop_rasters(
country_codes = "COM",
dest_dir = file.path(tf, "01_data", "1b_rasters", "pop_raster")
)
#> ✔ Population raster files successfully processed.
# Extract urban extent raster
extract_afurextent(
dest_dir = file.path(tf, "01_data", "1b_rasters", "urban_extent")
)
#> ℹ Extracting raster file to /tmp/RtmpioKZmp/test_env/01_data/1b_rasters/urban_extent...
#> Warning: cannot remove file '/tmp/RtmpioKZmp/test_env/01_data/1b_rasters/urban_extent/__MACOSX', reason 'Directory not empty'
#> ✔ Raster file successfully extracted to: /tmp/RtmpioKZmp/test_env/01_data/1b_rasters/urban_extent/afurextent.asc
#> [1] "/tmp/RtmpioKZmp/test_env/01_data/1b_rasters/urban_extent/afurextent.asc"
urban_raster <- terra::rast(
file.path(tf, "01_data", "1b_rasters",
"urban_extent", "afurextent.asc"))
pop_raster <- terra::rast(
file.path(tf, "01_data", "1b_rasters", "pop_raster",
"com_ppp_2020_constrained.tif")
)
adm2_sf <- sf::read_sf(
file.path(tf, "01_data", "1c_shapefiles",
"district_shape.gpkg"))
country_sf <- sf::st_union(adm2_sf)
predictors <- create_prediction_data(
country_code = "COM",
country_shape = country_sf,
pop_raster = pop_raster,
ur_raster = urban_raster,
adm2_shape = adm2_sf,
cell_size = 5000,
output_dir = file.path(
tf, "03_outputs/3a_model_outputs"
)
)
#> ℹ Processing shapefiles and grids...
#> ✔ Processed shapefiles and grids.
#>
#> ℹ Processing population raster...
#> ✔ Processed population raster.
#>
#> ℹ Processing urban-rural raster...
#> ✔ Processed urban-rural raster.
#>
#> ℹ Extracting data from rasters onto grid...
#> ✔ Extracted data from rasters onto grid.
#>
#> ℹ Creating grided data with predictors...
#> ✔ Created grided data with predictors.
#>
#> ✔ Predictors data created and saved at /tmp/RtmpioKZmp/test_env/03_outputs/3a_model_outputs/com_predictor_data.rds
# }